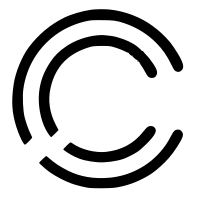
* feat: PR 리뷰 ai 정적 분석 기능 추가 * fix: CreateAiPullComment의 반환값을 *AiPullComment로 변경 * fix: review_test 모킹 타입 오류 수정 * fix: ai interface server config 파일 설정 추가 및 ai interface server 요청 방식 변경
53 lines
1.6 KiB
Go
53 lines
1.6 KiB
Go
package issues_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"code.gitea.io/gitea/models/db"
|
|
repo_model "code.gitea.io/gitea/models/repo"
|
|
"code.gitea.io/gitea/models/unittest"
|
|
user_model "code.gitea.io/gitea/models/user"
|
|
_ "github.com/mattn/go-sqlite3"
|
|
"github.com/stretchr/testify/assert"
|
|
|
|
ai_comment "code.gitea.io/gitea/models/issues"
|
|
)
|
|
|
|
func TestCreateAiPullComment(t *testing.T) {
|
|
|
|
assert := assert.New(t)
|
|
assert.NoError(unittest.PrepareTestDatabase())
|
|
|
|
pull := unittest.AssertExistsAndLoadBean(t, &ai_comment.Issue{ID: 2})
|
|
doer := unittest.AssertExistsAndLoadBean(t, &user_model.User{ID: 1})
|
|
repo := unittest.AssertExistsAndLoadBean(t, &repo_model.Repository{ID: pull.RepoID})
|
|
content := "this code is sh**"
|
|
treePath := "/src/ddd"
|
|
newAiComment, err := ai_comment.CreateAiPullComment(db.DefaultContext, &ai_comment.CreateAiPullCommentOption{
|
|
Doer: doer,
|
|
Pull: pull,
|
|
Repo: repo,
|
|
Content: content,
|
|
TreePath: treePath,
|
|
})
|
|
assert.NoError(err)
|
|
assert.EqualValues(newAiComment.Content, content)
|
|
assert.EqualValues(newAiComment.TreePath, treePath)
|
|
assert.EqualValues(newAiComment.PosterID, doer.ID)
|
|
assert.EqualValues(newAiComment.PullID, pull.ID)
|
|
|
|
}
|
|
|
|
func TestDeleteAiPullRequest(t *testing.T) {
|
|
|
|
|
|
assert.NoError(t, unittest.PrepareTestDatabase())
|
|
|
|
comment := unittest.AssertExistsAndLoadBean(t, &ai_comment.AiPullComment{ID: 2})
|
|
assert.NoError(t, ai_comment.DeleteAiPullCommentByID(db.DefaultContext, comment.ID))
|
|
unittest.AssertNotExistsBean(t, &ai_comment.AiPullComment{ID: comment.ID})
|
|
|
|
assert.NoError(t, ai_comment.DeleteAiPullCommentByID(db.DefaultContext, unittest.NonexistentID))
|
|
unittest.CheckConsistencyFor(t, &ai_comment.AiPullComment{})
|
|
}
|